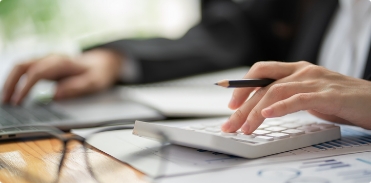
Introduction to Programming in Python
Course Content from ZyanteThis course provides an introduction to both the principles and the practice of programming, using a subset of Python 3. Materials and activities include: animations, learning questions, web-based programming windows, and web-based challenge activities. The course covers basic programming constructs (branches, loops, functions) to advanced topics such as inheritance, exceptions, and plotting.
This Course Includes:
- Proctored Exams
- 48 hours grading turn-around
- Live technical and student support
- Free transcription to your destination school
- 150+ partner college and universities with direct articulation
- Self Paced
- Computer Science
- Content by Zyante
No reviews have been submitted for Introduction to Programming in Python
After completing this course, students will be able to:
- Use standard input and output, and understand common syntax errors
- Declare and initialize variables with valid identifiers
- Develop programs that branch based on user input
- Combine loops and arrays/lists, and develop programs with multiple arrays/lists
- Write a function/method, then return from a function/method and parameterize a function
- Initialize class variables with class constructor
- Create derived and abstract classes
- Write a recursive function
- Use binary search, O notation, and algorithm analysis
Topic Number |
Topic Title |
Subtopics |
1 |
Introduction to Python 3 |
|
2 |
Variables and Expressions |
|
3 |
Types |
|
4 |
Branching |
|
5 |
Loops |
|
6 |
Functions |
|
7 |
Strings |
|
8 |
Lists and Dictionaries |
|
9 |
Classes |
|
10 |
Exceptions |
|
11 |
Modules |
|
12 |
Files |
|
13 |
Inheritance |
|
14 |
Recursion |
|
15 |
Plotting |
|
16 |
Searching and Sorting Algorithms |
|
17 |
Final Exam |
|
StraighterLine suggests, though does not require, that students take Pre-Calculus or its equivalent before enrolling in this course.
StraighterLine does not apply letter grades. Students earn a percentage score. A passing percentage is 70% or higher.
If you have chosen a Partner College to award credit for this course, your final grade will be based upon that college’s grading scale. Only passing scores will be considered by Partner Colleges for an award of credit.
There are a total of 1000 points in the course:
Topic |
Assessment |
Points Available |
1 | Chapter 1: Introduction to Python 3 | 31 |
2 | Chapter 2: Variables and Expressions | 31 |
3 | Chapter 3: Types | 31 |
4 | Chapter 4: Branching | 31 |
5 | Chapter 5: Loops | 31 |
6 | Chapter 6: Functions | 31 |
7 | Chapter 7: Strings | 31 |
8 | Chapter 8: Lists and Dictionaries | 31 |
8 | Midterm Exam | 204 |
9 | Chapter 9: Classes | 31 |
10 | Chapter 10: Exceptions | 31 |
11 | Chapter 11: Modules | 31 |
12 | Chapter 12: Files | 31 |
13 | Chapter 13: Inheritance | 31 |
14 | Chapter 14: Recursion | 31 |
15 | Chapter 15: Plotting | 31 |
16 | Chapter 16: Searching and Sorting Algorithms | 31 |
17 | Final Exam | 300 |
Total |
1000 |