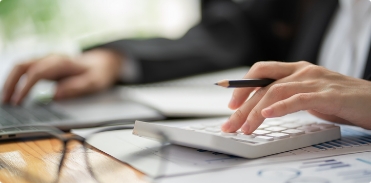
Introduction to Programming in Java
Course Content from ZyanteThis course is focused on the interactive learning of programming in Java foundations and emphasizes a solid understanding of memory. From the moment variables are introduced, the material shows via animations how variables exist and are updated in memory. The continued showing of memory helps clarify challenging topics like pass-by-copy/reference parameters, object creation, garbage collection, and more. Furthermore, the material includes emphasis on disciplined program development, including incremental development, modular development, and testing/debugging.
This Course Includes:
- Proctored Exams
- 48 hours grading turn-around
- Live technical and student support
- Free transcription to your destination school
- 150+ partner college and universities with direct articulation
- Self Paced
- Computer Science
- Content by Zyante
No reviews have been submitted for Introduction to Programming in Java
After completing this course, students will be able to:
- Use standard input and output, and understand common syntax errors
- Declare and initialize variables with valid identifiers
- Develop programs that branch based on user input
- Combine loops and arrays/lists, and develop programs with multiple arrays/lists
- Write a function/method, then return from a function/method and parameterize a function
- Initialize class variables with class constructor
- Create derived and abstract classes
- Write a recursive function
- Use binary search, O notation, and algorithm analysis
Topic Number |
Topic Title |
Subtopics |
1 |
Introduction to Java |
|
2 |
Variables / Assignments |
|
3 |
Branches |
|
4 |
Loops |
|
5 |
Arrays |
|
6 |
User-Defined Methods |
|
7 |
Objects and Classes |
|
8 |
Memory Management |
|
9 |
Input / Output |
|
10 |
Inheritance |
|
11 |
Recursion |
|
12 |
Exceptions |
|
13 |
Generics |
|
14 |
Collections |
|
15 |
GUI |
|
16 |
JavaFX |
|
17 |
Searching and Sorting Algorithms |
|
18 |
Final Exam |
|
StraighterLine suggests, though does not require, that students take Pre-Calculus or its equivalent before enrolling in this course.
StraighterLine does not apply letter grades. Students earn a percentage score. A passing percentage is 70% or higher.
If you have chosen a Partner College to award credit for this course, your final grade will be based upon that college’s grading scale. Only passing scores will be considered by Partner Colleges for an award of credit.
There are a total of 1000 points in the course:
Topic |
Assessment |
Points Available |
1 | Chapter 1: Introduction to Java | 29 |
2 | Chapter 2: Variables / Assignments | 29 |
3 | Chapter 3: Branches | 29 |
4 | Chapter 4: Loops | 29 |
5 | Chapter 5: Arrays | 29 |
6 | Chapter 6: User-Defined Methods | 29 |
7 | Chapter 7: Objects and Classes | 29 |
8 | Chapter 8: Memory Management | 29 |
9 | Chapter 9: Input / Output | 29 |
9 | Midterm Exam | 207 |
10 | Chapter 10: Inheritance | 29 |
11 | Chapter 11: Recursion | 29 |
12 | Chapter 12: Exceptions | 29 |
13 | Chapter 13: Generics | 29 |
14 | Chapter 14: Collections | 29 |
15 | Chapter 15: GUI | 29 |
16 | Chapter 16: JavaFX | 29 |
17 | Chapter 17: Searching and Sorting Algorithms | 29 |
18 | Final Exam | 300 |
Total |
1000 |